공공데이터를 쓰기 위해서는 공공데이터포털에서 인증키를 발급받아야한다.
https://www.data.go.kr/index.do
공공데이터 포털
국가에서 보유하고 있는 다양한 데이터를『공공데이터의 제공 및 이용 활성화에 관한 법률(제11956호)』에 따라 개방하여 국민들이 보다 쉽고 용이하게 공유•활용할 수 있도록 공공데이터(Datase
www.data.go.kr
나는 총 2개를 공부용으로 신청했다.
더보기
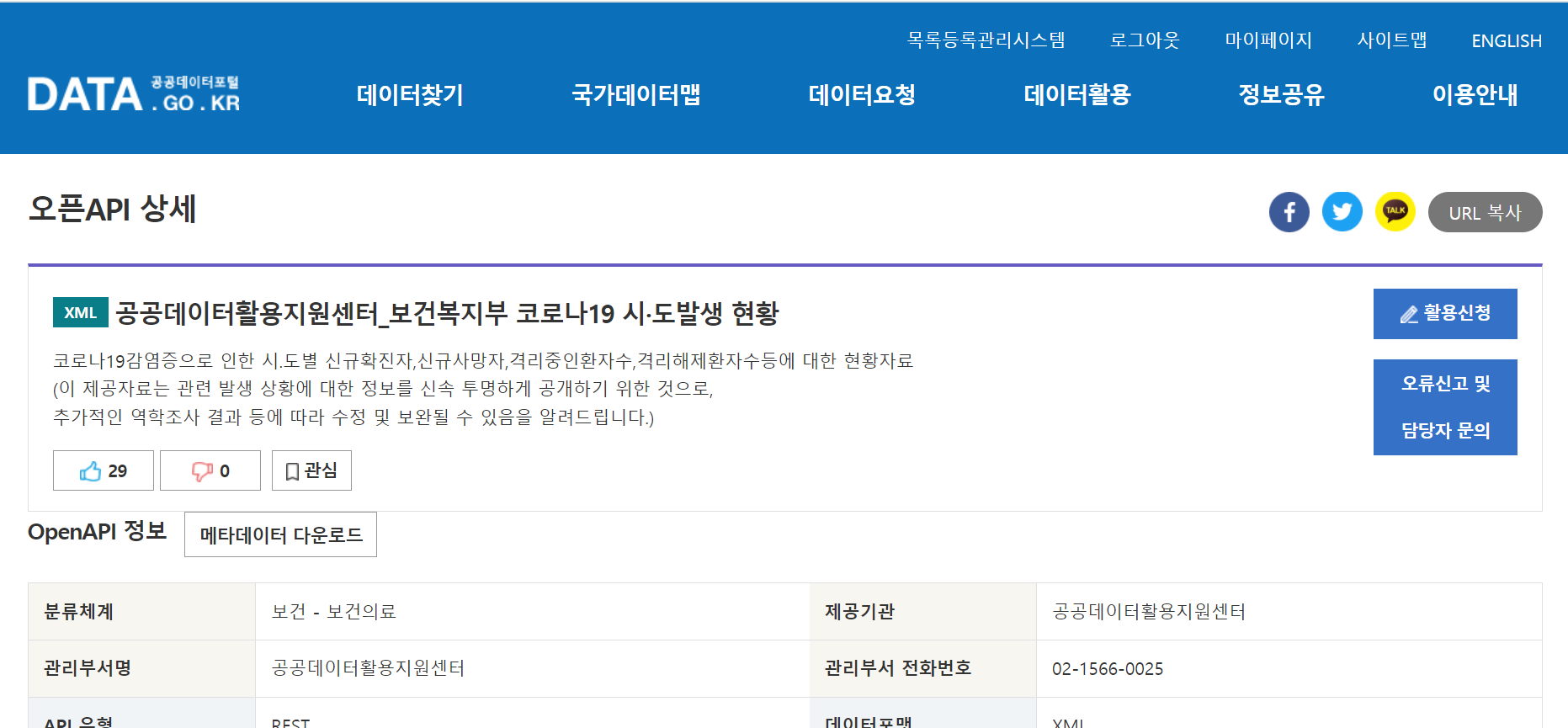
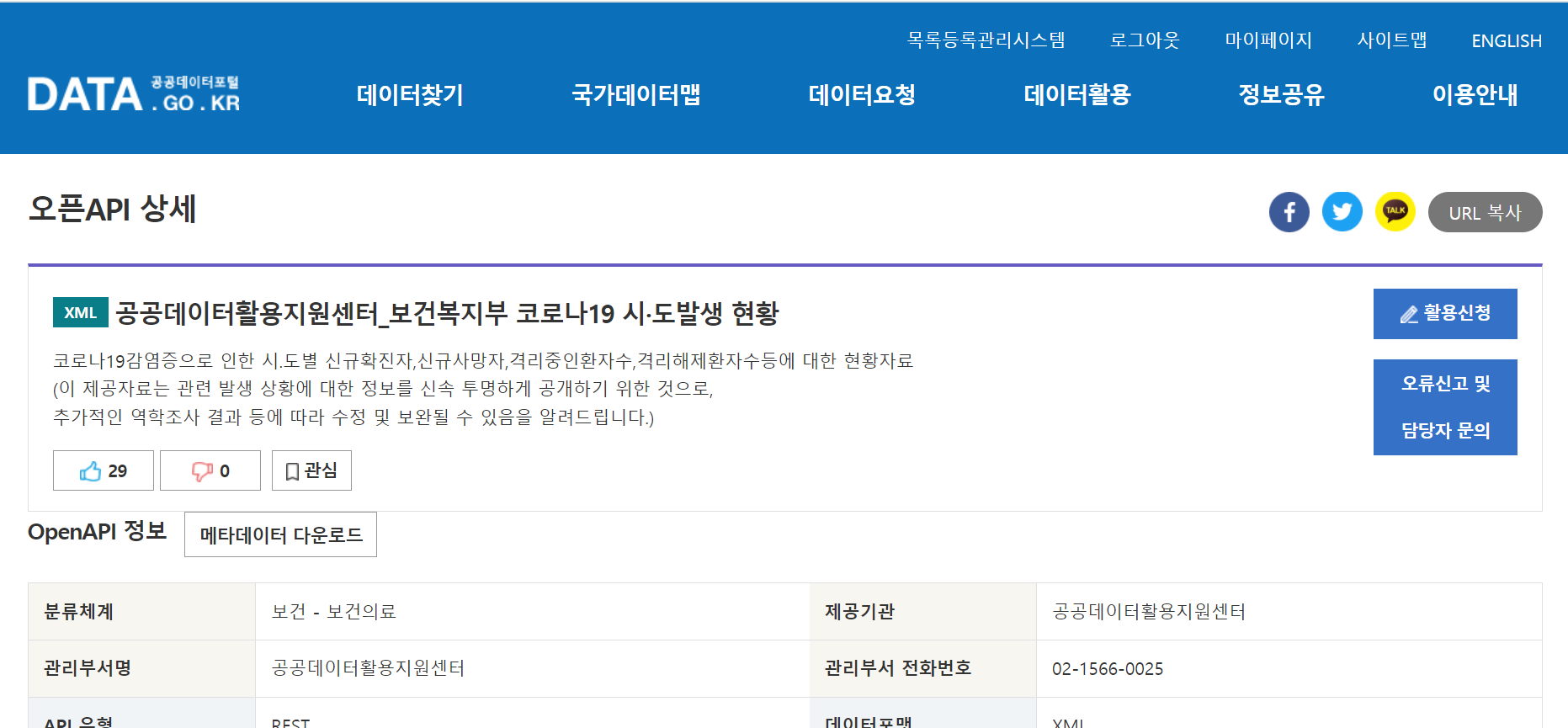
옆에 활용신청을 눌러서 신청할 수 있다.
공공 API 등의 데이터를 XML, JSON 문서를 String 형태로 받기 위한 자바 클래스
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import org.json.JSONObject;
import org.json.XML;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Service;
@Service("common.apiSerializer")
public class APISerializer {
private final Logger logger = LoggerFactory.getLogger(getClass());
// 공공 API 등의 데이터를 XML,JSON 문서를 String 형태로 받기
public String receiveToString(String spec) throws Exception {
String result = null;
HttpURLConnection conn = null;
try {
conn = (HttpURLConnection) new URL(spec).openConnection();
BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream(), "UTF-8"));
StringBuilder sb = new StringBuilder();
String s;
while ((s = br.readLine()) != null) {
sb.append(s);
}
result = sb.toString();
} catch (Exception e) {
logger.error(e.toString());
throw e;
} finally {
if (conn != null) {
try {
conn.disconnect();
} catch (Exception e2) {
}
}
}
return result;
}
// 공공 API등의 XML 데이터를 String 형태의 JSON으로 변환하여 받기
public String receiveXmlToJson(String spec) throws Exception {
String result = null;
try {
String s = receiveToString(spec);
/*
* <dependency> <groupId>org.json</groupId> <artifactId>json</artifactId>
* <version>20210307</version> </dependency>
*
*/
JSONObject job = XML.toJSONObject(s);
result = job.toString();
} catch (Exception e) {
// logger.error(e.toString());
throw e;
}
return result;
}
}
HttpURLConnection 에 요청변수(Request Parameter)와 함께 서비스 URL을 넣으면
출력결과를 얻을 수 있다. 사이트 밑에 샘플 코드도 제공되어 있으므로 참고하시면됩니다.
자바에서 받아온 후 AJAX를 통해 서버에 요청하면 jsp에 뿌려주는 방식으로 진행했다.
@RequestMapping(value = "covid", method = RequestMethod.GET, produces = "application/json; charset=utf-8")
public String covid(@RequestParam String date) throws Exception {
String result = null;
int numOfRows = 20;
int pageNo = 1;
String serviceKey = "개개인의서비스키";
String spec = "http://openapi.data.go.kr/openapi/service/rest/Covid19/getCovid19SidoInfStateJson";
spec += "?serviceKey=" + serviceKey + "&numOfRows=" + numOfRows + "&pageNo=" + pageNo +
"&startCreateDt=" + date + "&endCreateDt=" + date;
result = apiSerializer.receiveXmlToJson(spec);
return result;
}
@RequestMapping에 produces를 통해 "application/json; charset=utf-8" 을 명시해야 깨지지 않음
사이트에서 안내된 파라미터와 샘플데이터를 보고 맞춰서 넣어주어야한다. (대/소문자도 틀리면안됨)
function ajaxFun(url, method, query, dataType, fn) {
$.ajax({
type:method,
url:url,
data:query,
dataType:dataType,
success:function(data){
fn(data);
},
error:function(e) {
console.log(e.responseText);
}
});
}
$(function(){
$("#btnCovid").click(function(){
var url="${pageContext.request.contextPath}/parse/covid";
var date = "20211213";
var query = "date=" + date;
var fn = function(data) {
printCovid(data);
};
ajaxFun(url, "get", query, "json", fn);
});
function printCovid(data) {
var out="<h3>코로나 발생 현황</h3><hr>";
console.log(data);
$.each(data.response.body.items.item, function(index, item) {
if(index == 0 ) {
out += "기준일시 : " + item.stdDay + "<br>";
}
out += item.gubun
+ " - 전일대비 확진자 증가수 : " + item.incDec
+ ", 누적확진자 수 : " + item.defCnt
+ "<br>";
});
$("#resultLayout").html(out);
}
});
'쌍용강북교육센터 > 12월 + 2022년 1월' 카테고리의 다른 글
Tomcat 포트번호 변경 및 문자 인코딩(UTF-8) (0) | 2021.12.26 |
---|---|
MariaDB 설치시 유의사항 및 간단한 쿼리 (0) | 2021.12.24 |
[Spring] AOP(Aspect Oriented Programming) 관점 지향 프로그래밍 (0) | 2021.12.21 |
1216_10자리의 임시 비밀번호 만들기 (0) | 2021.12.21 |
1213_highcharts 라이브러리 활용한 차트만들기 (0) | 2021.12.13 |