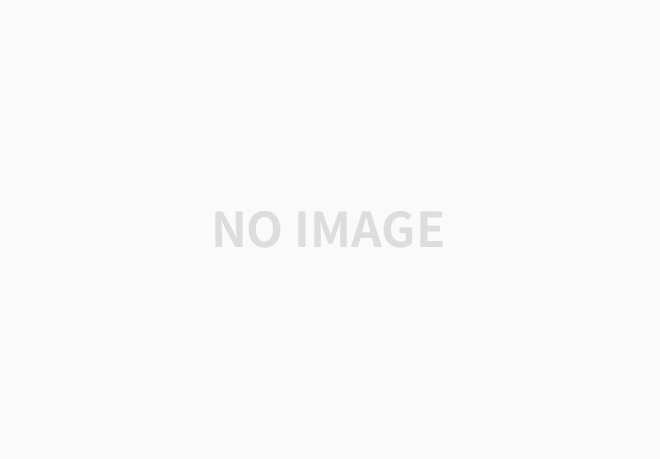
'정보' 카테고리의 다른 글
File-Tree-Generator 프로젝트 구조 트리 생성기 (0) | 2025.03.21 |
---|---|
유용한 정보: favicon generator, adobe color-wheel (0) | 2025.03.06 |
HtmlEditor 브라우저별 동작 차이 (feat. sencha htmlEditor) (0) | 2025.01.10 |
EXCEL 문자열 ERROR 시 (0) | 2024.12.11 |
이모지 EMOJI 정규식 REGEX (0) | 2024.12.11 |