Ex005_Runnable : 1초마다 찍는 스레드
더보기

package threadEx;
import java.util.Calendar;
public class Ex005_Runnable {
public static void main(String[] args) {
Thread t = new Thread(new MyThread5());
t.start();
}
}
class MyThread5 implements Runnable {
@Override
public void run() {
String str;
while (true) {
Calendar cal = Calendar.getInstance();
str = String.format("%tF %tT", cal, cal);
System.out.println(str);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
Thread.sleep(1000); 괄호 안에는 millisecond 단위이므로 1000이면 1초를 뜻한다.

terminate를 누르지 않으면 계속 시간이 찍힌다.
Ex006_Runnable : 윈도우창을 활용한 1초마다 시간이 바뀌게 하는 예제 [JFrame 상속 후 Runnable 인터페이스 구현]
더보기
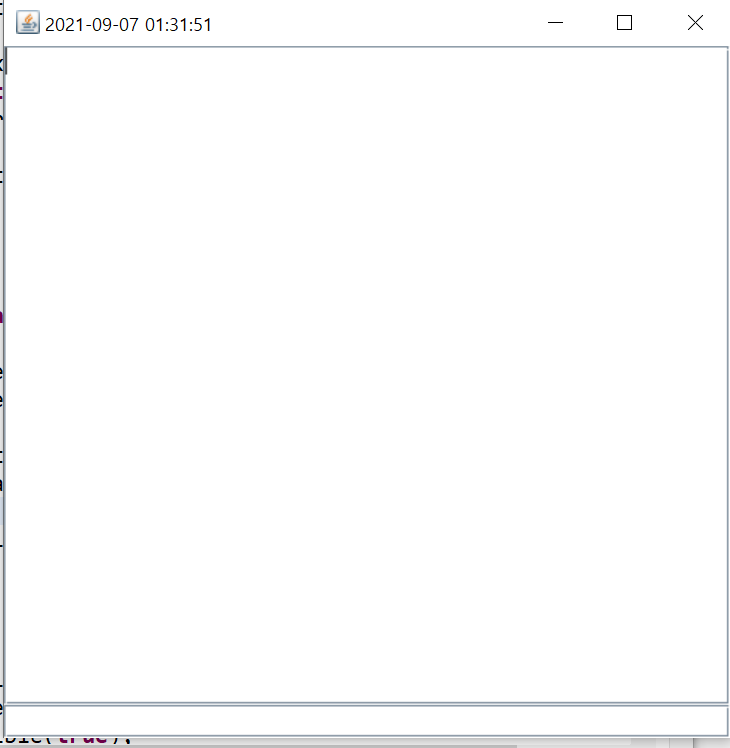

package threadEx;
import java.awt.BorderLayout;
import java.util.Calendar;
import javax.swing.JFrame;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
public class Ex006_Runnable {
public static void main(String[] args) {
ChatForm cf = new ChatForm();
Thread t = new Thread(cf);
t.start();
}
}
class ChatForm extends JFrame implements Runnable {
private static final long serialVersionUID = -5166274042184939576L;
private JTextField tf = new JTextField();
private JTextArea ta = new JTextArea();
public ChatForm() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JScrollPane sp = new JScrollPane(ta);
add(sp, BorderLayout.CENTER);
add(tf, BorderLayout.SOUTH);
setTitle("채팅...");
setSize(500,500);
setVisible(true);
}
@Override
public void run() {
String s;
while(true) {
try {
Calendar cal = Calendar.getInstance();
s = String.format("%tF %tT", cal, cal);
setTitle(s);
} catch (Exception e) {
}
}
}
}
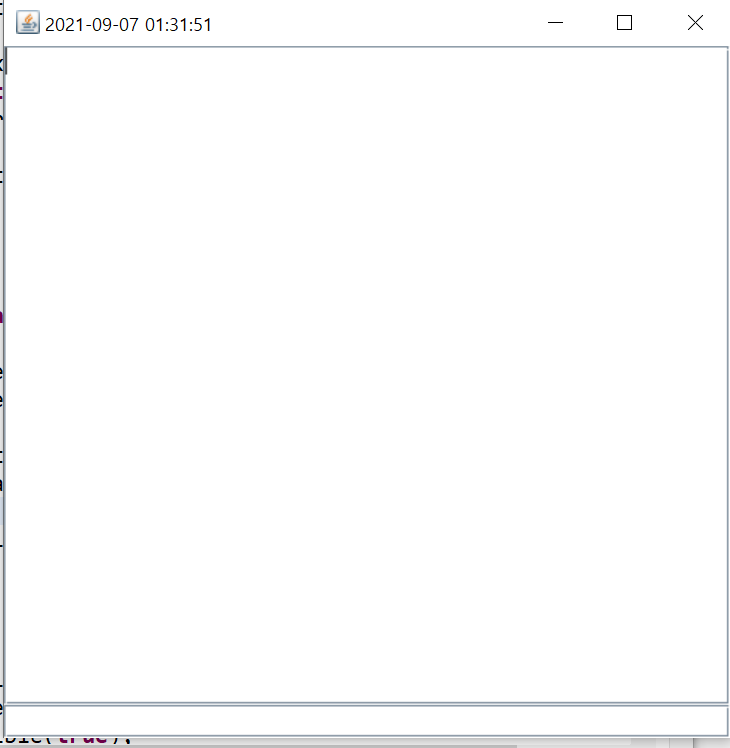

위 코드를 실행하면 이렇게 따로 윈도우창이 뜨고 창 제목이 시스템 시간에 따라 1초씩 찍히는 것을 확인할 수 있다.
'쌍용강북교육센터 > 9월' 카테고리의 다른 글
0903_Java : Thread Priority 스레드 우선순위 (0) | 2021.09.07 |
---|---|
0903_Java : Daemon Thread (0) | 2021.09.07 |
0903_Java : java.lang.Thread 스레드 클래스 (0) | 2021.09.07 |
0902_데이터 모델링 및 설계 (0) | 2021.09.03 |
0902_Java : JDBC : 자바로 SQLPlus와 비슷한 프로그램 짜기 (0) | 2021.09.02 |