클래스(class)
- class는 객체를 생성하기 위한 템플릿이다.
- 클래스는 데이터와 이를 조작하는 코드를 하나로 추상화한다.
- ES 2015(ECMAScript 6) 이전까지는 비슷한 종류의 객체를 만들어내기 위해 생성자를 사용했다.
- ES 2015(ECMAScript 6) 에서 도입된 클래스는 생성자의 기능을 대체한다. class 표현식을 사용하면, 생성자와 같은 기능을 하는 함수를 훨씬 더 깔끔한 문법으로 정의할 수 있다.
개요
- class는 "특별한 함수"이다.
- 함수를 함수 표현식과 함수 선언으로 정의할 수 있듯이, class도 class 표현식과 class 선언 두 가지 방법을 이용하여 정의할 수 있다.
- 클래스는 함수로 호출될 수 없다.
- 클래스 선언은 let과 const처럼 블록 스코프에 선언한다.
- 클래스의 메소드 안에서 super 키워드를 사용할 수 있다.
- 함수 선언과 클래스 선언의 중요한 차이점은 함수 선언의 경우 호이스팅이 일어나지만, 클래스 선언은 호이스팅이 일어나지 않는다.
※ 클래스는 함수, 객체가 아니다.
class Person {
console.log('hello');
}
위 결과는 에러 : Unexpected token
class Person {
prop1:1,
prop2:2
}
위 결과는 에러 : Unexpected token
클래스 몸체
- 클래스 body는 중괄호 {}로 묶여 있는 안쪽 부분으로 메소드나 constructor와 같은 class 멤버를 정의한다.
- 클래스의 본문(body)은 strict mode에서 실행된다. 즉, 여기에 적힌 코드는 성능 향상을 위해 더 엄격한 문법이 적용된다.
Constructor(생성자)
- constructor 메소드는 class로 생성된 객체를 생성하고 초기화하기 위한 특수한 메소드이다.
- "constructor"라는 이름을 가진 특수한 메소드는 클래스 안에 한 개만 존재할 수 있다. 클래스에 여러 개의 constructor메소드가 존재하면 SyntaxError가 발생한다.
- constructor는 부모 클래스의 constructor를 호출하기 위해 super 키워드를 사용할 수 있다.
클래스 정의 : class 표현식
- class 표현식은 class를 정의하는 또 다른 방법이다.
- class 표현식은 이름을 가질 수도 있고, 갖지 않을 수도 있다.
- 이름을 가진 class표현식의 이름은 클래스 body의 loval scope에 한해 유효하다.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
// 클래스
class User {
// 생성자 : 생성자는 클래스안에서 constructor 라는 이름으로 정의한다.
constructor({name, age}) {
this.name = name;
this.age = age;
}
// 메소드를 만드는 문법 그대로 사용하면 메소드가 자동으로 User.prototype 에 저장된다.
msg() {
return '안녕하세요. 제 이름은 ' + this.name + '입니다.';
}
}
const obj = new User({name:'호호호', age:20});
console.log( obj.msg() );
console.log( typeof User) // function
console.log( typeof User.prototype.constructor ); // function
console.log( typeof User.prototype.msg ); // function
console.log( obj instanceof User ); // true
</script>
</head>
<body>
<h3>클래스</h3>
</body>
</html>
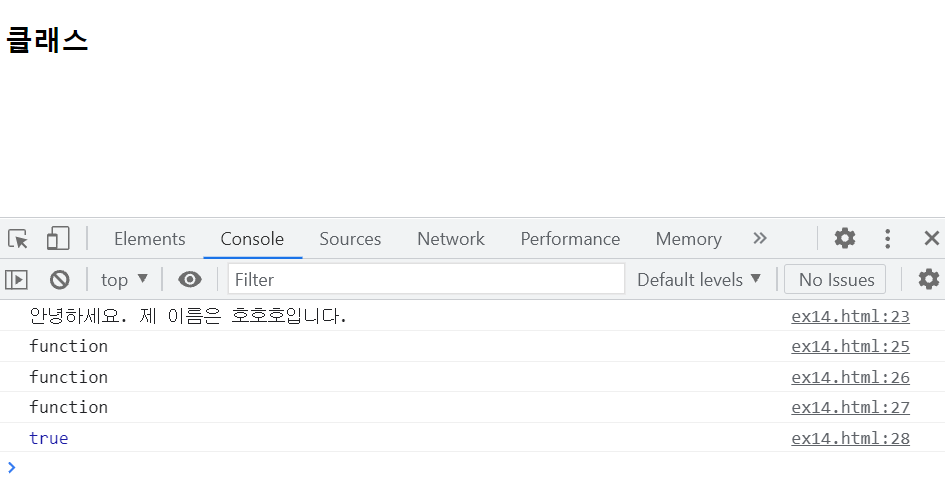
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
class User {
add(x, y) {
return x+y; // 프로퍼티가 없으므로 this를 쓰면 안된다.
}
subtract(x, y) {
return x-y;
}
}
const obj = new User();
console.log( obj.add(10, 5) );
</script>
</head>
<body>
<h3>클래스</h3>
</body>
</html>
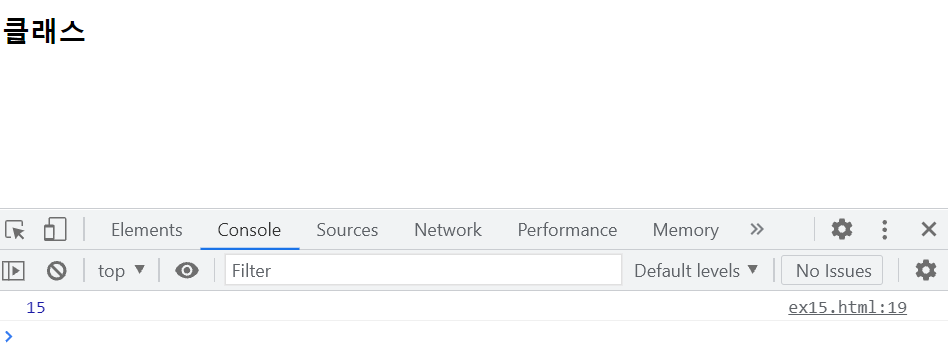
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
const methodName = 'sayName';
class User {
constructor(name, age) {
this.name = name;
this.age = age;
}
// 임의의 표현식을 []로 둘러쌓아 메소드 이름으로 사용
[methodName]() {
return `안녕 나는 ${this.name}이야. `; // 템플릿 리터널
}
}
const obj = new User('나나나', 10);
console.log( obj.sayName() );
</script>
</head>
<body>
<h3>클래스</h3>
</body>
</html>

클래스의 getter 혹은 setter를 정의하고 싶을 때는 메소드 이름 앞에 get 또는 set을 붙여주면 된다.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
class User {
constructor() {
this._name = "";
}
get name() {
return this._name;
}
set name(newName) {
this._name = newName;
}
}
const obj = new User();
obj.name = '홍길동'; // 속성처럼 부름. 메소드를 프로퍼티처럼 사용
console.log( obj.name );
</script>
</head>
<body>
<h3>클래스 - getter, setter</h3>
</body>
</html>

<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
class Rect {
constructor(height, width) {
this.height = height;
this.width = width;
}
// getter
get area() {
return this.calc();
}
// 메소드
calc() {
return this.height * this.width;
}
}
var obj = new Rect(10, 5);
console.log( obj.area );
console.log( obj.calc() ); // 괄호가 있어야지 해당 메소드를 호출
</script>
</head>
<body>
<h3>클래스</h3>
</body>
</html>
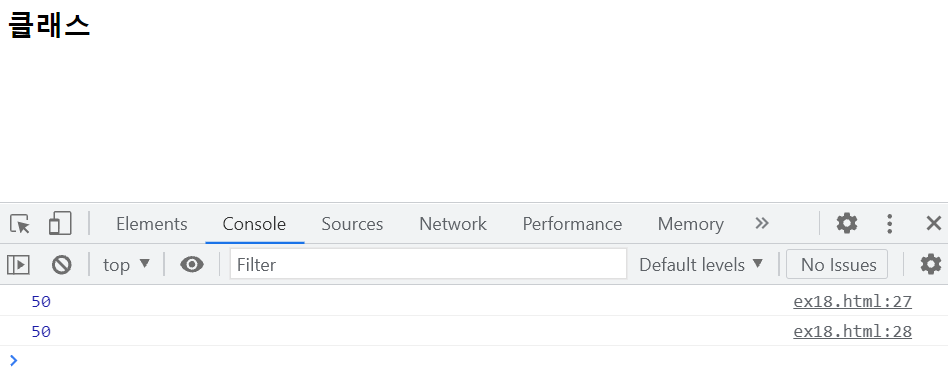
ex19 클래스 예제
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
/*
class Rect {
// 생성자
constructor(h, w) {
this.height = h;
this.width = w;
}
}
var obj = new Rect(10, 5);
console.log(obj.height, obj.width);
*/
class Rect {
// 메소드
area(h, w) {
this.height = h;
this.width = w;
return this.height * this.width; // 생성자뿐만 아니라 메소드에서도 속성 추가 가능
}
}
var obj = new Rect();
var a = obj.area(5, 7);
console.log(a);
console.log(obj.height, obj.width);
console.log(obj);
</script>
</head>
<body>
<h3>클래스</h3>
</body>
</html>
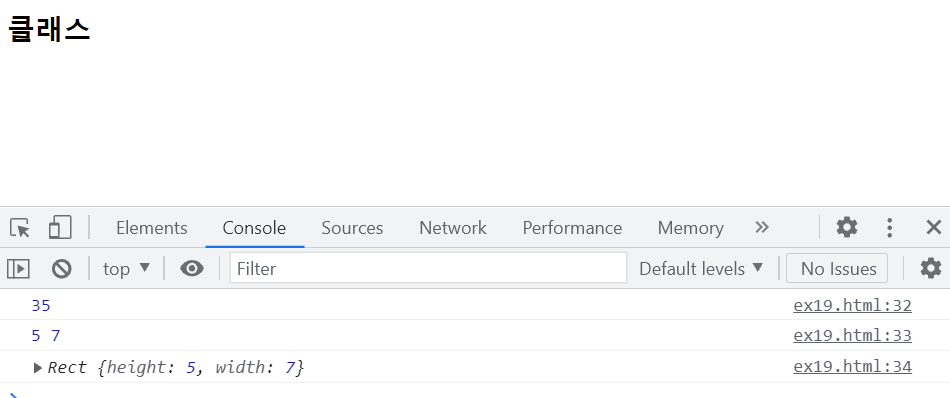
클래스 - 상속 예제
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
class Car {
constructor(name) {
this.name = name;
}
speed() {
console.log(`${this.name}은 시속 80 입니다.`);
}
color() {
console.log(this.name +'은 검정색입니다.')
}
}
class Taxi extends Car {
constructor(name) {
super(name); // 상위 클래스의 생성자 호출
}
speed() { // 자바에서 override
super.speed(); // 상위 클래스의 메소드 호출
console.log(this.name+'은 시속 100 입니다.')
}
}
var obj = new Taxi('그랜저');
console.log(obj.name);
obj.speed();
obj.color();
</script>
</head>
<body>
<h3>클래스 - 상속</h3>
</body>
</html>
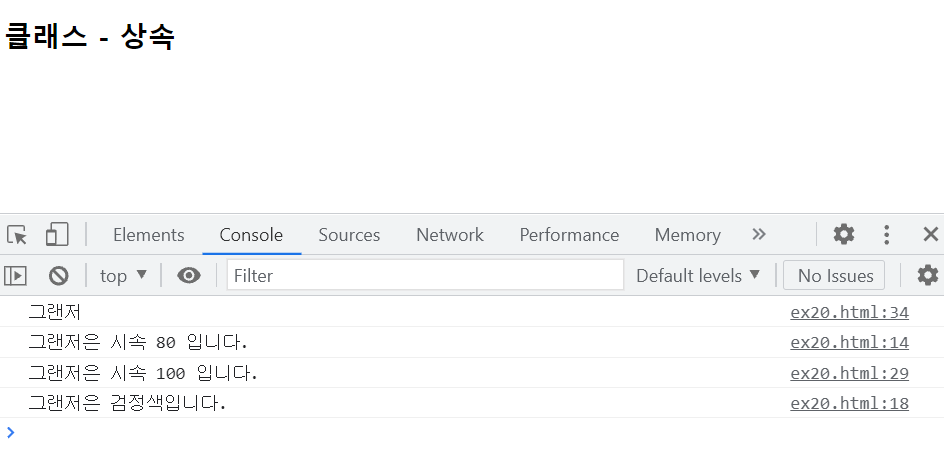
'쌍용강북교육센터 > 10월' 카테고리의 다른 글
1005_JavaScript : 쪽지보낼 사람 선택하기 (0) | 2021.10.05 |
---|---|
1005_JavaScript : 회원가입 유효성 검사 (0) | 2021.10.05 |
1005_JavaScript : 게시판 전체선택 체크박스 (0) | 2021.10.05 |
1005_JavaScript : <form> 요소 (0) | 2021.10.05 |
1001_JavaScript : 프로토타입 Prototype (0) | 2021.10.03 |