파일업로드를 위한 <form> 태그
<form> 태그의 enctype 속성
- <form> 태그 안에 정의된 요청 파라미터를 클라이언트에서 서버로 전송할 때 인코딩 방법을 지정한다.
- HTTP 헤더의 Content-Type 을 지정하여 서버에 어떤 종류의 데이터가 전송되는지를 전달한다.
- method="post"인 경우에만 이 속성은 유효하다.
- 속성 값
1) application/x-www-form-urlencoded
기본 enctype 속성으로 요청 파라미터는 key1=value1&key2=value2 형태로 나열된다.
공백은 +, 영숫자가 아닌 문자는 %HH(문자코드의 16진수 2자리)로 인코딩하여 전송한다.
이 content type은 바이너리 데이터(파일 등) 전송에서는 사용할 수 없다.
2) multipart/form-data
문자 인코딩을 하지 않고 전송되며, 파일을 전송할 때 사용한다.
여러 형태의 데이터 (파일 내용, 텍스트 입력 값 등)가 동시에 전달되며 각 데이터는 boumdary=...(경계정보) 뒤에 붙은 문자열을 이용하여 구분한다.
3) text/plain
공백이 +문자로 변환되며 특수 문자를 인코딩 하지 않는다.
예제 (ch09)
더보기
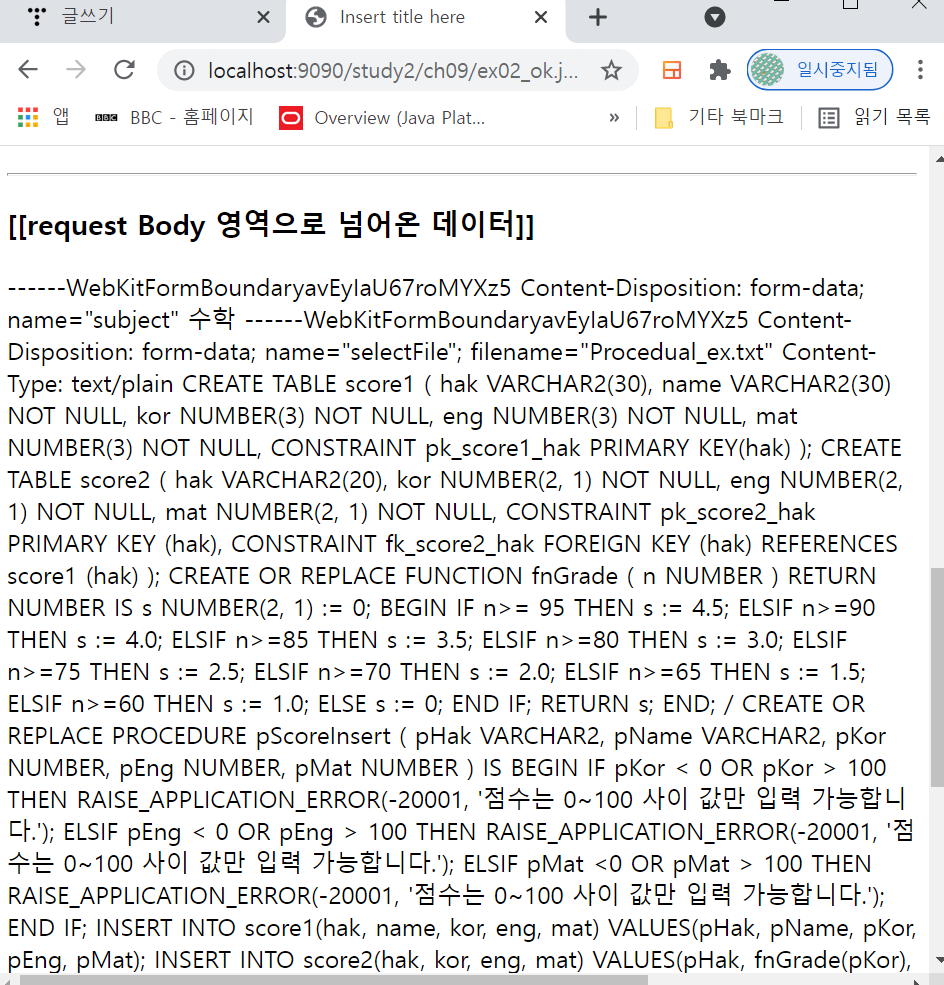
ex01
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ page trimDirectiveWhitespaces="true"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
</head>
<body>
<h3>파일 업로드</h3>
<%--
- form 태그에서 enctype을 생략하면 기본이 application/x-www-form-urlencoded
- application/x-www-form-urlencoded 속성 값은 파라미터를 주소형식으로 인코딩하여 전송
파라미터는 "이름1=값1&이름=값2" 형태로 body영역에 실어서 전송된다.
- application/x-www-form-urlencoded 속성 값에서 파일을 서버로 전송하면 파일 이름만 전송 된다.
- enctype 속성은 method가 post인 경우만 유효
--%>
<form action="ex01_ok.jsp" method="post" >
<p> 제목 : <input type="text" name="subject"> </p>
<p> 파일 : <input type="file" name="selectFile"> </p>
<p>
<button type="submit">등록하기</button>
</p>
</form>
</body>
</html>
ex01_ok
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ page trimDirectiveWhitespaces="true"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<%
request.setCharacterEncoding("utf-8");
// request.getParameter()는 문자열만 전달 받는다.
String subject = request.getParameter("subject");
// 파일은 enctype="application/x-www-form-urlencoded" 에서는 이름만 전송 받는다.
String selectFile = request.getParameter("selectFile");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
</head>
<body>
<h3>요청 받은 정보</h3>
<p>제목 : <%= subject %> </p>
<p>파일 : <%=selectFile %> </p>
</body>
</html>
위 예제를 통해서 파일을 전달 받지 못하는 것을 알 수 있다. 파일의 이름만을 받았음.
ex02
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ page trimDirectiveWhitespaces="true"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
</head>
<body>
<h3>파일 업로드</h3>
<%--
* form 태그에서 enctype이 multipart/form-data인 경우
- 문자 인코딩을 하지 않고 전송하며, 파일을 전송할 때 사용
- 피일의 내용도 전송된다.
* enctype 속성은 method가 post인 경우만 유효
--%>
<form action="ex02_ok.jsp" method="post" enctype="multipart/form-data">
<p> 제목 : <input type="text" name="subject"> </p>
<p> 파일 : <input type="file" name="selectFile"> </p>
<p>
<button type="submit">등록하기</button>
</p>
</form>
</body>
</html>
ex02_ok
<%@page import="java.net.URLDecoder"%>
<%@page import="java.io.InputStream"%>
<%@page import="java.util.Enumeration"%>
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ page trimDirectiveWhitespaces="true"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<%
request.setCharacterEncoding("utf-8");
// enctype="multipart/form-data"로 넘어온 파라미터는 request.getParameter()로 받을 수 없다.
// String subject = request.getParameter("subject");
// String selectFile = request.getParameter("selectFile");
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
</head>
<body>
<h3>요청 받은 정보</h3>
<%
String contentType = request.getContentType();
out.print("<p>contentType : " + contentType + "</p>");
out.print("<hr>");
out.print("<h3>[[헤더 정보들]]....</h3>");
Enumeration<String> e = request.getHeaderNames();
while(e.hasMoreElements()) {
String name = e.nextElement();
String value = request.getHeader(name);
out.print("<p>" + name + " : " + value + "</p>");
}
out.print("<hr>");
out.print("<h3>[[request Body 영역으로 넘어온 데이터]]</h3>");
InputStream is = request.getInputStream();
byte[] buf = new byte[2048];
int size;
String str;
while( (size = is.read(buf)) != -1) {
// enctype = "application/x-www-form-urlencoded" 인 경우
// str = new String(buf, 0, size);
// str = URLDecoder.decode(str, "utf-8");
// enctype = "nultipart/form-data" 인 경우
str = new String(buf, 0, size, "utf-8");
out.print("<p>" + str + "</p>");
}
out.print("<hr>");
%>
</body>
</html>
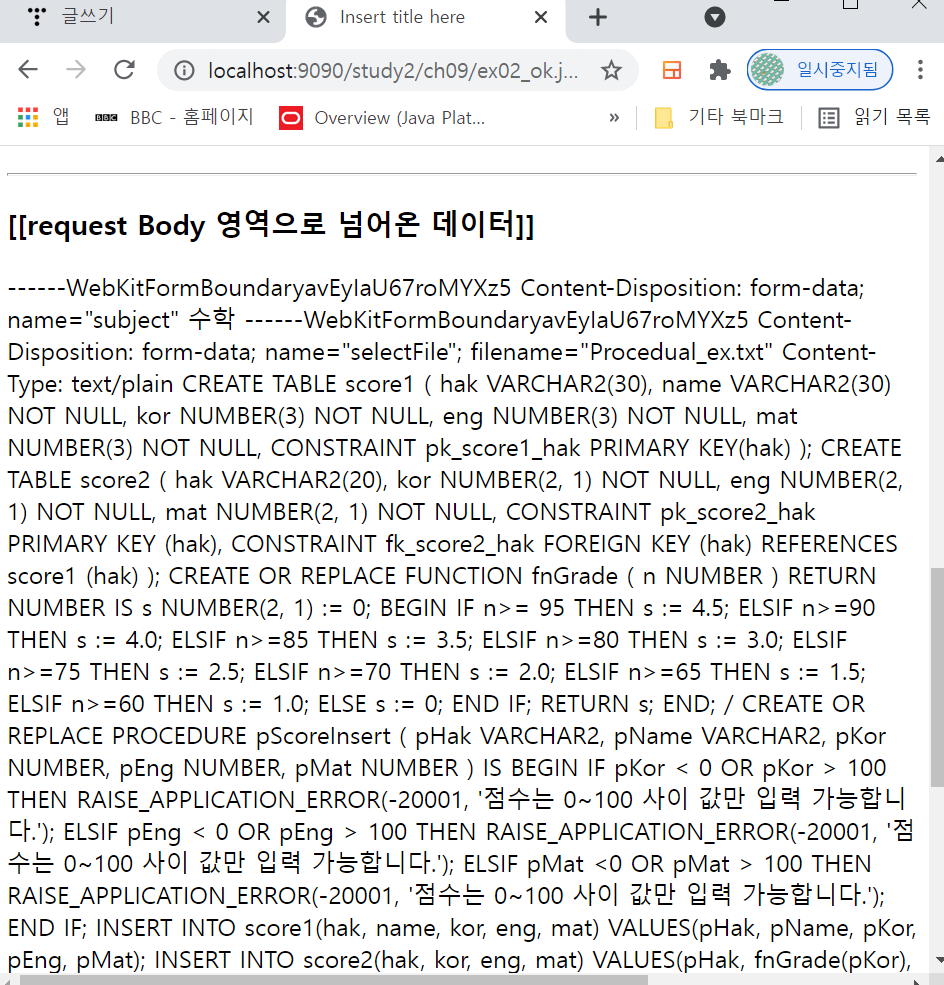
파일이 넘어왔음을 알 수 있다.
cos.jar을 이용한 업로드 (권장하지 않음)
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ page trimDirectiveWhitespaces="true"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
</head>
<body>
<h3>파일 업로드</h3>
<%--
* cos.jar를 이용한 업로드
http://servlets.com
--%>
<form action="ex03_ok.jsp" method="post" enctype="multipart/form-data">
<p> 제목 : <input type="text" name="subject"> </p>
<p> 파일 : <input type="file" name="selectFile"> </p>
<p>
<button type="submit">등록하기</button>
</p>
</form>
</body>
</html>
<%@page import="com.oreilly.servlet.multipart.DefaultFileRenamePolicy"%>
<%@page import="com.oreilly.servlet.MultipartRequest"%>
<%@page import="java.io.File"%>
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ page trimDirectiveWhitespaces="true"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<%
request.setCharacterEncoding("utf-8");
// String root = pageContext.getServletContext().getRealPath("/"); 도 가능
String root = session.getServletContext().getRealPath("/");
String pathname = root + "uploads" + File.separator + "pds";
File f= new File(pathname);
if(! f.exists() ) {
f.mkdirs();
}
String encType = "utf-8"; // 클라이언트가 보낸 정보의 인코딩
int maxFileSize = 5*1024*1024; // 최대 업로드 용량(5MB)
MultipartRequest mreq = null;
// request, 파일저장경로, 파일최대크기, 인코딩, 중복파일명보호
mreq = new MultipartRequest(request, pathname, maxFileSize, encType,
new DefaultFileRenamePolicy() );
// 제목
String subject = mreq.getParameter("subject");
// 서버에 저장된 파일 이름
String saveFilename = mreq.getFilesystemName("selectFile");
// 클라이언트가 올린 파일 이름
String originalFilename = mreq.getOriginalFileName("selectFile");
// 파일 크기
long fileSize = 0;
File file = mreq.getFile("selectFile"); // 업로드된 파일에 대한 File 객체
if(file != null) {
fileSize = file.length();
}
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
</head>
<body>
<h3>파일 업로드 결과 : cos.jar</h3>
<p> 제목 : <%=subject%> </p>
<p> 클라이언트가 올린 파일 이름 : <%=originalFilename %></p>
<p> 서버에 저장된 파일 이름 : <%=saveFilename %></p>
<p> 파일 크기 : <%=fileSize %>byte.</p>
</body>
</html>
'쌍용강북교육센터 > 10월' 카테고리의 다른 글
1027_jQuery : 선택자 Selectors (0) | 2021.10.28 |
---|---|
1027_jQuery : jQuery, Core (0) | 2021.10.28 |
1021_JSP/Servlet : 세션 Session (0) | 2021.10.25 |
1021_JSP/Servlet : 쿠키 Cookie (0) | 2021.10.25 |
1018~1019_JSP/Servlet : 자유게시판 만들기 :: ★중요★흐름, 파라미터생각해보기 (0) | 2021.10.20 |