연산자란 ?
연산자는 변수나 상수에 미리 약속된 연산을 행하기 위해 사용되며, 연산되는 항의 개수 따라 이항 연산자(binary operator), 단항 연산자(unary operator)등으로 나누어진다.
연산자
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
var a, b, c;
// +, -, *, /, %, ++, --
c = 10 / 0;
console.log(c); // Infinity : 무한대를 나타내는 숫자
if(c === Infinity) {
console.log('0으로 나누었습니다.');
}
a = 2;
b = 10;
c = a ** b; // 거듭 제곱
console.log(c); // 1024
c = 2 ** 3 ** 2; // 2 ** (3 ** 2)과 동일. 우결합성
console.log(c); // 512
// c = -2 ** 2; // 에러. 모호한 표현은 허용하지 않음
// 문자열 결합
a = "seoul";
b = "korea";
c = a + b ;
console.log(c);
a = 10;
b = "5";
c = a + b ; // 숫자 + 문자 => 문자 + 문자 => 문자
console.log(c); // 105
</script>
</head>
<body>
<h3>연산자</h3>
</body>
</html>
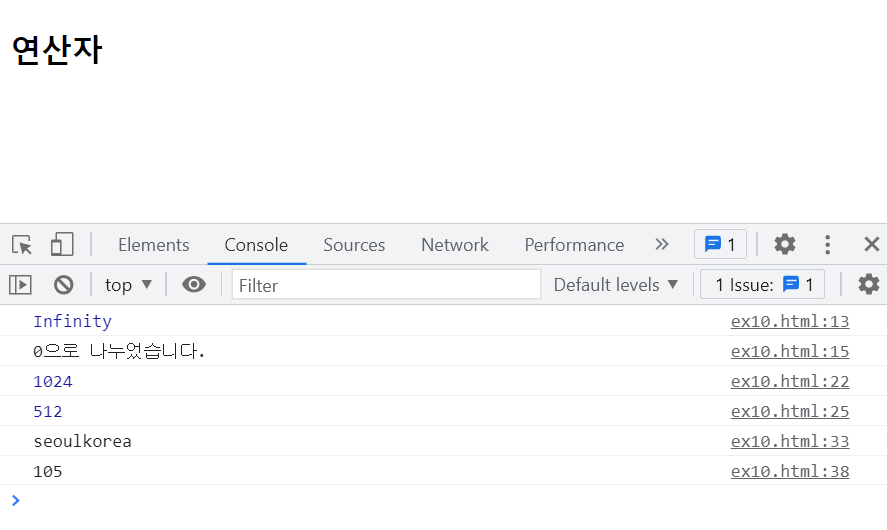
연산자 - 비교 연산자
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
var a, b;
a = '123';
b = 123;
console.log(a == b); // true
console.log(a === b); // false(동치). 두 값을 비교할 때는되도록이면 ===을 사용할 것을 권장
console.log(a != b); // false
console.log(a !== b); // true(동치가 아닐 경우 참)
</script>
</head>
<body>
<h3>연산자 - 비교 연산자</h3>
</body>
</html>
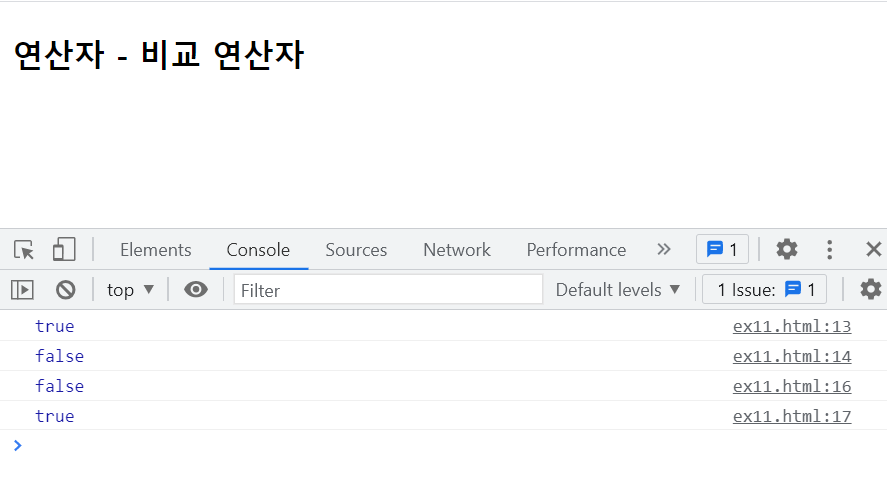
연산자 - 논리
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="icon" href="data:;base64,iVBORw0KGgo=">
<script type="text/javascript">
var a = "";
// 값이 "", 0, null, undefined, NaN 등은 거짓이다.
if(a) {
console.log("참...")
} else {
console.log("거짓...")
}
a = 0;
if(a) {
console.log("true...")
} else {
console.log("false...")
}
// 값이 있으면 true 로 생각함.
a = "html" && "css";
console.log(a); // css
a = "html" && true;
console.log(a); // true
a = false && "css";
console.log(a); // false
a = "html" || "css";
console.log(a); // html
a = "html" || true;
console.log(a); // html
a = false || "css";
console.log(a); // css
a = 0 || "css";
console.log(a); // css
a = 10 || "css";
console.log(a); // 10
</script>
</head>
<body>
<h3>연산자 - 논리</h3>
</body>
</html>

논리연산은 단축연산을 기억할 것
'쌍용강북교육센터 > 9월' 카테고리의 다른 글
0924_Javascript : 기본내장함수 parseInt, parseFloat (0) | 2021.09.25 |
---|---|
0924_Javascript : ex13 숫자를 입력받아 javascript로 연산하여 결과를 출력 (0) | 2021.09.25 |
0924_Javascript : 리터널 Liternal (0) | 2021.09.25 |
0924_Javascript : 상수 Constant (0) | 2021.09.25 |
0924_Javascript : 데이터타입 및 형 (0) | 2021.09.25 |